Best 7 Common API Vulnerabilities in TypeScript-Based ERP (With Fixes)
Introduction to API Vulnerabilities in TypeScript-Based ERP
APIs are the backbone of modern ERP (Enterprise Resource Planning) systems. They facilitate seamless communication between different modules, third-party integrations, and external systems. However, API vulnerabilities in TypeScript-based ERP systems can expose sensitive business data, disrupt operations, and lead to security breaches.
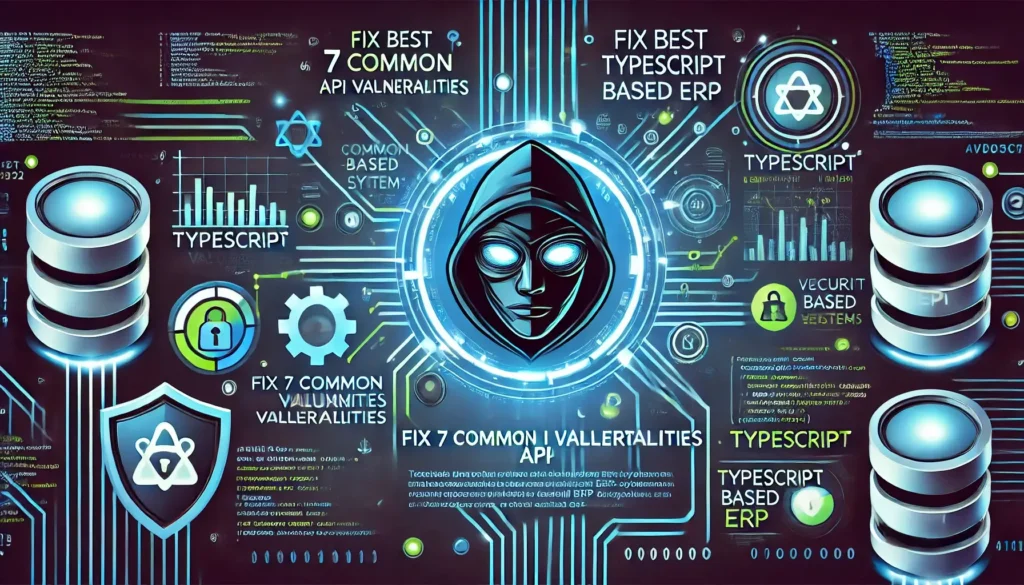
In this blog, we’ll dive deep into the common API vulnerabilities in TypeScript-based ERP systems, explore practical coding examples, and provide mitigation techniques to secure APIs.
What Are API Vulnerabilities in ERP Systems?
API vulnerabilities refer to weaknesses in an API’s implementation or configuration that attackers can exploit. These vulnerabilities are particularly critical in ERP systems, as they manage core business processes like inventory, financial data, and customer information.
Top 7 API Vulnerabilities in TypeScript-Based ERP
1. Broken Object-Level Authorization
Attackers can exploit this vulnerability to access unauthorized objects.
Code Example: Vulnerable API Endpoint
app.get('/user/:id', async (req, res) => {
const user = await User.findById(req.params.id);
res.json(user);
});
Fix:
app.get('/user/:id', async (req, res) => {
if (req.user.id !== req.params.id && !req.user.isAdmin) {
return res.status(403).json({ error: 'Access denied' });
}
const user = await User.findById(req.params.id);
res.json(user);
});
2. Mass Assignment
Mass assignment vulnerabilities allow attackers to manipulate sensitive fields.
Code Example:
const user = new User(req.body);
await user.save();
Fix:
const user = new User({
name: req.body.name,
email: req.body.email,
});
await user.save();
3. Insecure Direct Object References (IDOR)
IDOR occurs when attackers can directly access sensitive objects (e.g., user IDs or files) without proper authorization.
Vulnerable Code Example:
// Fetching user profile without authorization checks
app.get('/user/:userId', async (req, res) => {
const user = await User.findById(req.params.userId);
res.json(user);
});
Secure Code Example:
// Validate that the user has the proper access to the requested resource
app.get('/user/:userId', async (req, res) => {
if (req.user.id !== req.params.userId && !req.user.isAdmin) {
return res.status(403).json({ error: 'Access denied' });
}
const user = await User.findById(req.params.userId);
res.json(user);
});
4. Improper Error Handling
Detailed error messages can expose sensitive system information to attackers.
Vulnerable Code Example:
app.post('/login', async (req, res) => {
try {
const user = await User.findOne({ email: req.body.email });
if (!user) throw new Error('User not found');
// Password validation here
} catch (err) {
res.status(500).send(err.message); // Leaks system details
}
});
Secure Code Example:
app.post('/login', async (req, res) => {
try {
const user = await User.findOne({ email: req.body.email });
if (!user) {
return res.status(400).json({ error: 'Invalid credentials' });
}
// Password validation here
} catch (err) {
console.error('Login error:', err); // Log error internally
res.status(500).json({ error: 'Something went wrong. Please try again.' });
}
});
5. Insufficient Logging and Monitoring
Without proper logging, you might miss critical events that indicate attacks.
Vulnerable Code Example:
// No logging
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Authentication logic
res.json({ message: 'Logged in' });
});
Secure Code Example:
const winston = require('winston'); // Using Winston for logging
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [new winston.transports.Console()],
});
app.post('/login', (req, res) => {
const { username } = req.body;
// Log login attempts
logger.info(`Login attempt by user: ${username}`);
// Authentication logic
res.json({ message: 'Logged in' });
});
6. Cross-Site Scripting (XSS) in RESTful APIs
XSS vulnerabilities occur when malicious scripts are injected and executed in a user’s browser.
Vulnerable Code Example:
app.get('/search', (req, res) => {
const query = req.query.q;
res.send(`<div>Search Results for: ${query}</div>`); // Vulnerable to XSS
});
Secure Code Example:
const escapeHtml = (unsafe: string) =>
unsafe
.replace(/&/g, '&')
.replace(/</g, '<')
.replace(/>/g, '>')
.replace(/"/g, '"')
.replace(/'/g, ''');
app.get('/search', (req, res) => {
const query = req.query.q;
res.send(`<div>Search Results for: ${escapeHtml(query)}</div>`); // XSS protected
});
7. Session Fixation
Session fixation occurs when an attacker tricks a user into using a session ID known to the attacker.
Vulnerable Code Example:
// Session ID is not regenerated after login
app.post('/login', (req, res) => {
req.session.userId = req.body.userId; // Keeps the old session ID
res.json({ message: 'Logged in' });
});
Secure Code Example:
// Regenerate session ID on login
app.post('/login', (req, res) => {
req.session.regenerate((err) => {
if (err) {
return res.status(500).json({ error: 'Failed to start a new session' });
}
req.session.userId = req.body.userId;
res.json({ message: 'Logged in' });
});
});
These examples demonstrate how to handle vulnerabilities effectively while maintaining clean and secure TypeScript code.
Coding Examples: Identifying and Fixing API Vulnerabilities
Example: Validating User Input
app.post('/api/login', (req, res) => {
const { username, password } = req.body;
if (!username || !password) {
return res.status(400).json({ error: 'Invalid input' });
}
// Proceed with authentication
});
Example: Securing API Keys
const apiKey = process.env.API_KEY || '';
if (req.headers['x-api-key'] !== apiKey) {
return res.status(403).json({ error: 'Unauthorized' });
}
How Our Free Tools Can Help Secure APIs
Using our free Website Security Scanner tool, you can analyze vulnerabilities in your APIs, including those in TypeScript-based ERP systems.
Below is a screenshot of our free tool’s homepage:
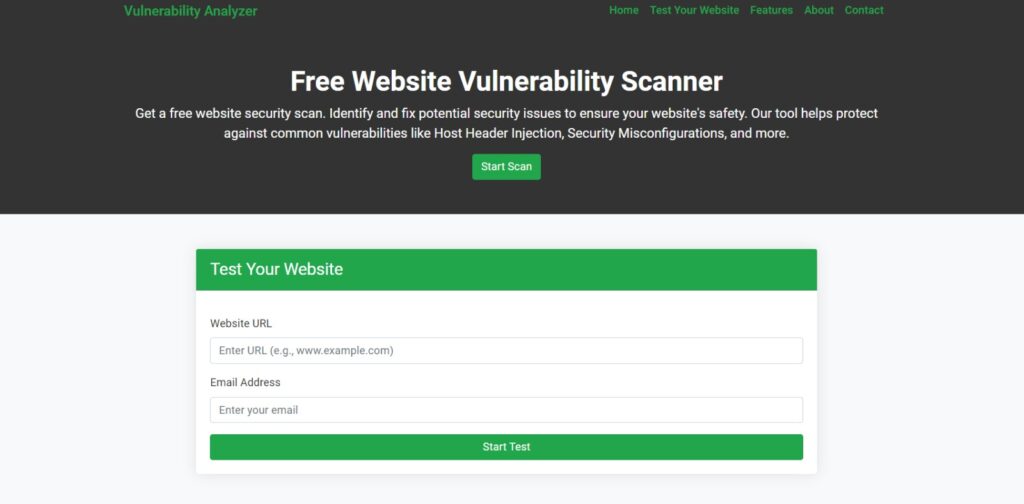
Additionally, here’s an example of a vulnerability assessment report generated using our tool to check website vulnerability:
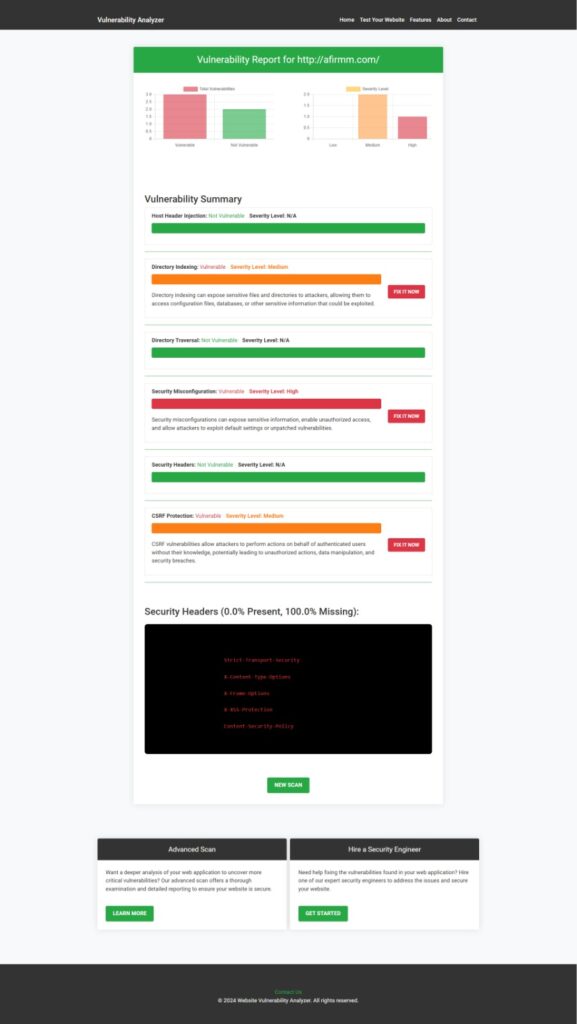
Related Resources
For additional insights, visit our blog:
- Prevent Host Header Injection in TypeScript
- Logging and Monitoring in TypeScript
- Session Fixation Attack in TypeScript
- How to Prevent XSS in RESTful APIs
- More Articles
Linking to Other Resources
Check out our article on Preventing Host Header Injection in OpenCart to learn how to secure your websites effectively.
Conclusion
Addressing API vulnerabilities in TypeScript-based ERP systems is crucial for maintaining data security and operational integrity. You can safeguard your APIs from potential threats by implementing the fixes provided in this guide and leveraging tools like our Website Security Checker.
Pingback: Best 7 Tips to Prevent Host Header Injection in TypeScript